Cirql (pronounced Circle) is a simple lightweight ORM and query builder for SurrealDB, providing fully type-safe queries and Zod powered parsing & validation. Unlike most query builders, Cirql's flexible nature leaves you with complete control over your queries, while still providing powerful APIs to query your database in a concise manner.
- ๐ Connect to SurrealDB over stateful WebSockets or stateless HTTP requests
- ๐ฆ Support for query batching & transactions
- โ๏ธ Zod-powered schema validation of query results
- ๐ Full TypeScript support with Zod schema inference
- ๐ Write flexible queries using the Query Writer API
- โ๏ธ Support for raw query strings and native Surreal functions
- ๐ฅ Works both in browser and backend environments
Cirql is still in early developmental stages. While you can use it for production applications, it may still lack specific features and edge cases. Feel free to submit feature requests or pull requests to add additional functionality to Cirql. We do ask you to please read our Contributor Guide.
While we try to prevent making any significant API changes, we cannot guarantee this.
The first step to use Cirql is to install the package from npm, together with a supported version of zod.
npm install cirql zod
You can read our documentation for information on how to use Cirql.
The following query fetches up to 5 organisations that are enabled and have the given user as a member. The result is parsed and validated using the provided Zod schema.
import { RecordSchema, select } from 'cirql';
import { z } from 'zod';
// Define your Zod schemas
const Organisation = RecordSchema.extend({
name: z.string(),
isEnabled: z.boolean(),
createdAt: z.string()
});
// Execute a select query
const organisations = await cirql.execute({
query: select()
.from('organisation')
.with(Organisation) // Specify the schema
.limit(5)
.where({
isEnabled: true,
members: any(userId)
})
});
Visit our Basic Usage guide for more examples.
We welcome any issues and PRs submitted to Cirql. Since we currently work on multiple other projects and our time is limited, we value any community help in supporting a rich future for Cirql.
Before you open an issue or PR please read our Contributor Guide.
- PNPM (npm i -g pnpm)
You can find the roadmap of intended features here.
The changelog of previous versions can be found here.
To run in live development mode, run pnpm dev
in the project directory. This will start the Vite development server.
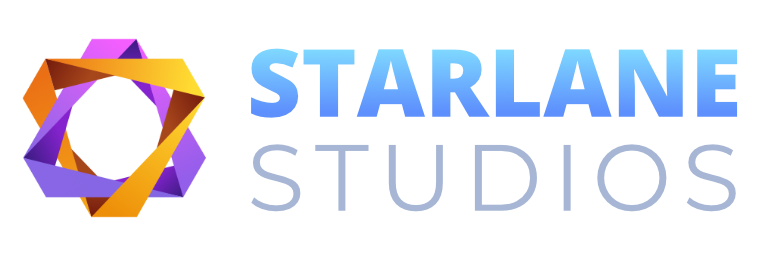
Cirql is built and maintained by Starlane Studios at no cost. If you would like to support our work feel free to donate to us โก
Cirql is licensed under MIT
Copyright (c) 2023, Starlane Studios