struct ContentView: View {
@State private var dayNight = "morning"
@State private var tab = 0
@State private var meals = "one"
var body: some View {
VStack(spacing: 20){
Text("Segment")
.font(.largeTitle)
Picker(selection: $dayNight, label: Text("DayPiker")) {
Text("Morning").tag("morning")
Text("Noon").tag("noon")
Text("Night").tag("night")
}.labelsHidden()
.pickerStyle(SegmentedPickerStyle())
Picker(selection: $tab, label: Text("")) {
Image(systemName: "sun.min").tag(0)
Image(systemName: "moon").tag(1)
}.pickerStyle(SegmentedPickerStyle())
Picker(selection: $meals, label: Text("")) {
Text("One").tag("one")
Text("Two").tag("two")
Text("Three").tag("three")
Text("More").tag("more")
}
.background(Color.yellow)
.cornerRadius(10)
.padding(.horizontal)
.pickerStyle(SegmentedPickerStyle())
Spacer()
}.padding()
}
}
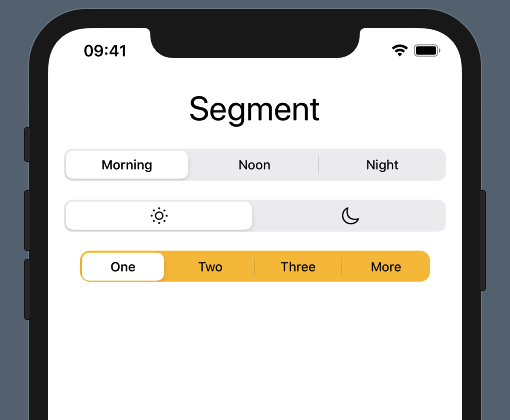
struct FontDesign: View {
var body: some View {
NavigationView {
VStack(spacing: 10) {
Text("Default font design")
.font(Font.system(size:30, design: .default))
Divider()
Text("Monospaced font design")
.font(Font.system(size:30, design: .monospaced))
Divider()
Text("Rounded font design")
.font(Font.system(size:30, design: .rounded))
Divider()
Text("Serif font design")
.font(Font.system(size:30, design: .serif))
Divider()
.navigationBarTitle(Text("Design"))
}.padding()
}
}
}
struct TextStyle: View {
var body: some View {
NavigationView {
VStack(spacing:10) {
Text("Large Title").font(.largeTitle)
Text("Title").font(.title)
Text("Headline").font(.headline)
Text("SubHeadline").font(.subheadline)
Text("Body").font(.body)
Text("Callout").font(.callout)
Text("Caption").font(.caption)
Text("FootNote").font(.footnote)
}
.navigationBarTitle(Text("Text Styles"))
}
}
}
struct WeightStyle: View {
var body: some View {
NavigationView {
VStack(spacing:10) {
Text("Ultralight").fontWeight(.ultraLight)
Text("Thin").fontWeight(.thin)
Text("Light").fontWeight(.light)
Text("Regular").fontWeight(.regular)
Text("Medium").fontWeight(.medium)
Text("SemiBold").fontWeight(.semibold)
Text("Bold").fontWeight(.bold)
VStack(spacing:10) {
Text("Heavy").fontWeight(.heavy)
Text("Black").fontWeight(.black)
}
}
.navigationBarTitle(Text("Weight"))
}
}
}
struct ContentView: View {
@State var selectedTab = 0
var body: some View {
TabView(selection:$selectedTab) {
TextStyle().tabItem {
Image(systemName: "textformat")
Text("Styles")
}.tag(0)
WeightStyle().tabItem {
Image(systemName: "bold")
Text("Weight")
}.tag(1)
FontDesign().tabItem {
Image(systemName: "text.cursor")
Text("Design")
}.tag(2)
}
}
}
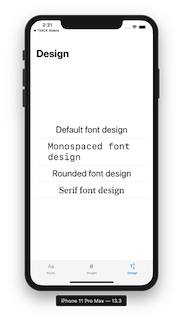
struct ContentView: View {
@State private var stepperValue = 1
@State private var values = [0,1]
var body: some View {
VStack(spacing:20) {
Text("Stepper")
.font(.largeTitle)
Stepper(value: $stepperValue) {
Text("Bound Stepper: \(stepperValue)")
}
Stepper(onIncrement: {
self.values.append(self.values.count)
}, onDecrement: {
self.values.removeLast()
}) {
Text("OnIncrement and OnDecrement")
}
ScrollView(.horizontal) {
HStack {
ForEach(values, id: \.self) { value in
Image(systemName: "\(value).circle.fill").font(.largeTitle)
.foregroundColor(.green)
}
}.padding()
}
Stepper(value: $stepperValue, in: 1...5) {
Text("Rating")
}.padding(.horizontal)
HStack {
ForEach(1...stepperValue, id: \.self) { star in
Image(systemName: "star.fill")
.foregroundColor(.yellow)
}
}
}.padding()
}
}
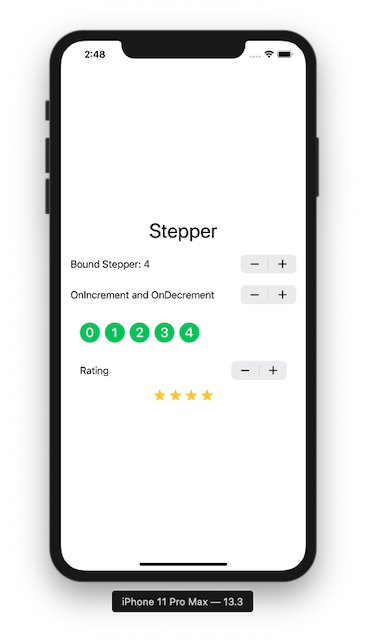
struct ContentView: View {
@State private var age = 18.0
@State private var sliderValue = 0.3
let ageFormatter: NumberFormatter = {
let numFormate = NumberFormatter()
numFormate.numberStyle = .spellOut
return numFormate
}()
var body: some View {
VStack(spacing:20){
Text("Slider")
Slider(value: $age, in: 1...100, step: 1)
Group {
Text("Age: ") + Text("\(ageFormatter.string(from: NSNumber(value: age))!)").foregroundColor(.green)
}
Slider(value: $sliderValue)
.padding(.horizontal)
.background(Color.yellow)
.cornerRadius(10)
.shadow(color: .gray, radius: 5)
.accentColor(Color.green)
Slider(value: $sliderValue)
.padding(.horizontal)
.background(Capsule().stroke(Color.orange, lineWidth: 2))
.accentColor(.orange)
Slider(value:$sliderValue)
.padding(.horizontal)
.background(Capsule().fill(Color.yellow))
.accentColor(.black)
HStack {
Image(systemName: "tortoise")
Slider(value:$sliderValue)
Image(systemName: "hare")
}.padding()
.foregroundColor(.green)
Spacer()
}.padding()
}
}
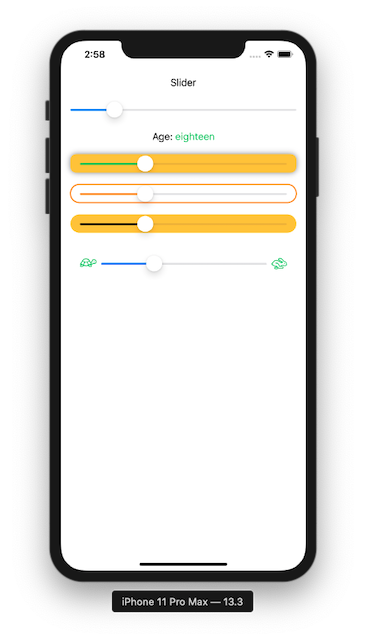
struct CircleCustomeTab: View {
var body: some View {
VStack(spacing: 15) {
Text("Circle")
Circle()
.fill(Color.orange)
.frame(width: 100)
Circle()
.stroke(Color.red, style: StrokeStyle(lineWidth: 4, dash: [9,5]))
//.stroke(Color.red, lineWidth: 5)
.frame(width:100)
Circle()
.foregroundColor(.green)
.frame(width: 100)
ZStack() {
Circle()
.stroke(Color.red,lineWidth: 10)
Circle()
.stroke(Color.yellow,lineWidth: 10)
.padding(20)
Circle()
.stroke(Color.black,lineWidth: 10)
.padding(40)
Circle()
.stroke(Color.purple,lineWidth: 10)
.padding(60)
}.frame(width:200, height:200)
ZStack {
Circle()
.fill(Color.secondary)
.frame(height:250)
Circle()
.fill(Color.secondary)
.frame(height:200)
Circle()
.fill(Color.secondary)
.frame(height:150)
Circle()
.fill(Color.secondary)
.frame(height:100)
}
}.padding()
}
}
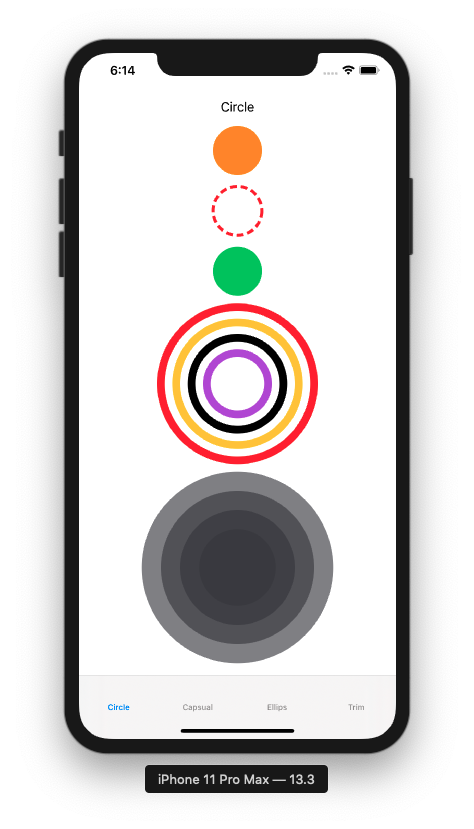
struct EllipeCustomView: View {
var body: some View {
VStack(spacing:20) {
Text("Ellips")
Ellipse()
ZStack {
Ellipse()
.stroke(Color.yellow, lineWidth: 30)
Ellipse()
.stroke()
}.padding()
ZStack {
Ellipse()
.strokeBorder(Color.pink,lineWidth: 30)
Ellipse()
.stroke()
}
Spacer()
}.padding()
}
}
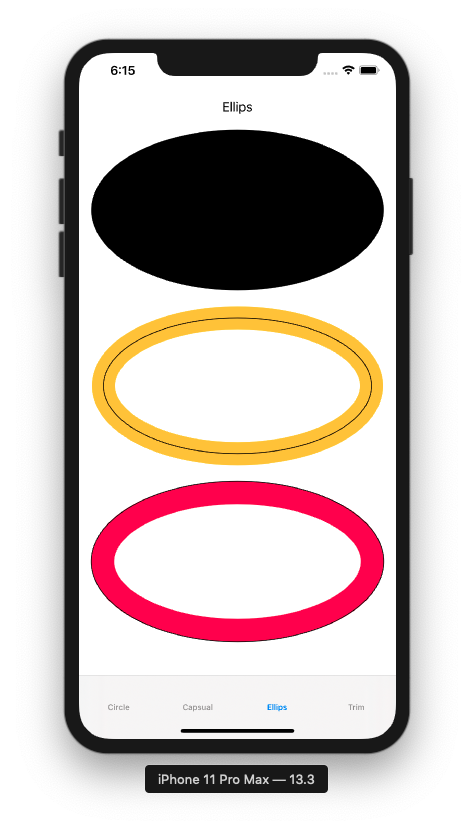
struct TripOutLine: View {
@State private var orangeCircleProgress:CGFloat = 0.9
@State private var greenCircleProgress:CGFloat = 0.3
private var percentage:Int {
get {
return Int(orangeCircleProgress * 100.0)
}
}
var body: some View {
VStack {
Text("Circle Shape")
.font(.largeTitle)
Text("Trim function")
.foregroundColor(.gray)
ZStack {
Circle()
.trim(from: 0.0, to: orangeCircleProgress)
.stroke(Color.orange, style: StrokeStyle(lineWidth: 40, lineCap: CGLineCap.round))
.frame(width:300)
.rotationEffect(.degrees(-90))
.overlay(
Text("\(percentage)%")
)
Circle()
.trim(from: 0.0, to: greenCircleProgress)
.stroke(Color.green, style: StrokeStyle(lineWidth: 40, lineCap: CGLineCap.round))
.frame(width:170)
.rotationEffect(.degrees(-90))
.overlay(
Text("\(percentage)%")
)
}
.padding()
Text("Completed Circle")
Slider(value: $orangeCircleProgress)
.padding(.horizontal)
.accentColor(.orange)
Slider(value: $greenCircleProgress)
.padding(.horizontal)
.accentColor(.green)
}.font(.title)
.padding()
}
}
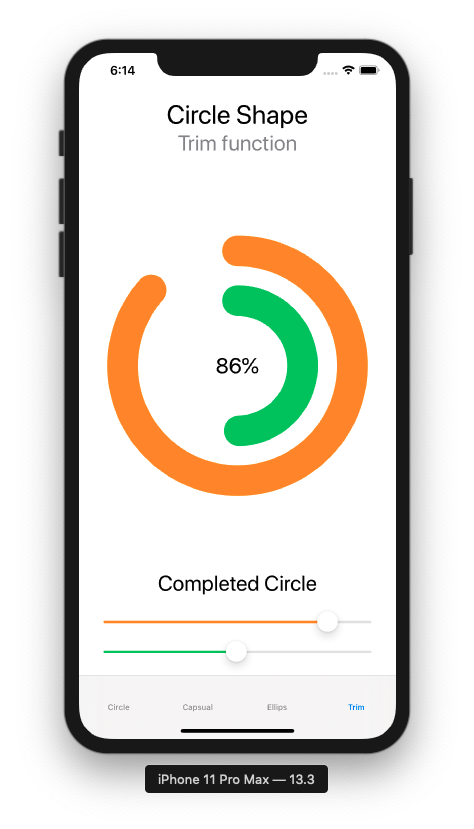
Action Sheet with isPresented
struct ContentView: View {
@State private var openActionsheet:Bool = false
var body: some View {
VStack(spacing:20) {
Text("Action Sheet")
Button(action: {
self.openActionsheet = true
}) {
Text("Open Action Sheet")
}
}.actionSheet(isPresented: $openActionsheet) { () -> ActionSheet in
ActionSheet(title: Text("Hello!"), message: Text("This is action sheet."), buttons: [
.default(Text("Action1"), action: {
// Action 1
}),
.default(Text("Action2"), action: {
// Action 2
}),
.destructive(Text("Red"), action: {
// Action 3
}),
.cancel({
// Cancel
})
])
}
}
}
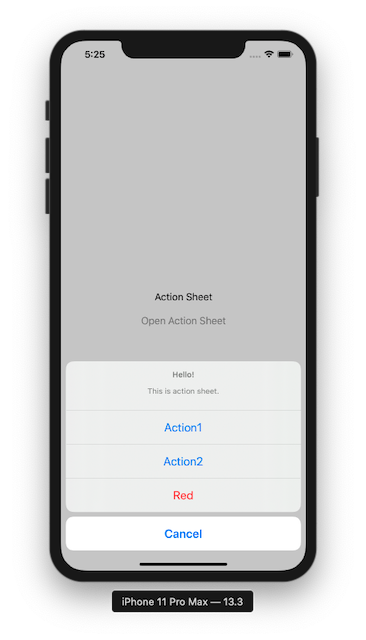
// Create Identifiable struct to hold action sheet data.
struct ActionSheetData:Identifiable {
var id = UUID()
var title:String
var message:String
}
struct customDataAction: View {
@State private var actionSheetData: ActionSheetData? = nil
var body: some View {
VStack(spacing:20) {
Text("Action Sheet")
.font(.largeTitle)
Text("Action with custom data")
.foregroundColor(.gray)
Button(action: {
self.actionSheetData = ActionSheetData(title: "Save", message: "Choose an option!")
}) {
Text("Open Action sheet")
}
}.actionSheet(item: $actionSheetData) { sheetData in
ActionSheet(title: Text(sheetData.title), message: Text(sheetData.message), buttons: [
.default(Text("Default Option"), action: {
//Option 1
}),
.destructive(Text("Destructive Option"), action: {
// Destructive option
}),
.cancel({
// Cancel
})
])
}
}
}
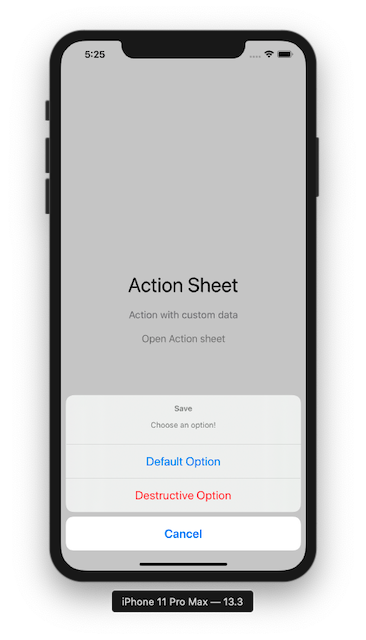
ContextMenu
struct ContentView: View {
var body: some View {
VStack(spacing:10) {
Text("Context Menu")
.font(.largeTitle)
Text("Introduction")
.foregroundColor(.gray)
Spacer()
HStack() {
Text("Get Help")
Spacer()
Image(systemName: "questionmark.diamond.fill")
.foregroundColor(.yellow)
.contextMenu {
Button(action: {}) {
Image(systemName: "eyedropper.full")
Text("Add Color")
}
Button(action: {}) {
Image(systemName: "sun.haze")
Text("Haze")
}
Button(action: {}) {
Image(systemName: "circle.lefthalf.fill")
Text("Change Constrast")
}
}
}.padding()
Spacer()
}.font(.title)
}
}
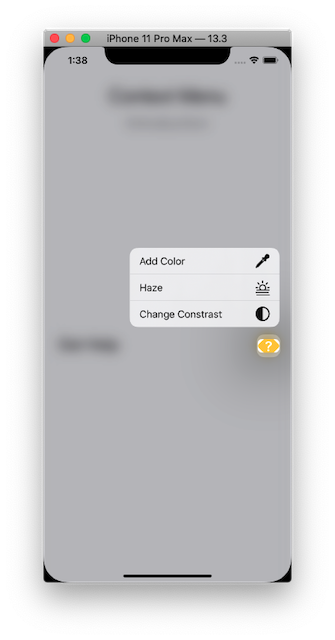
VStack(spacing: 20) {
Text("Offset")
.font(.largeTitle)
ZStack {
RoundedRectangle(cornerRadius: 10)
.frame(width: 200, height: 100)
.foregroundColor(.green)
.shadow(radius: 5)
.offset(x: -20, y: -10)
RoundedRectangle(cornerRadius: 10)
.frame(width: 200, height: 100)
.foregroundColor(.blue)
.shadow(radius: 5)
RoundedRectangle(cornerRadius: 10)
.frame(width: 200, height: 100)
.foregroundColor(.red)
.shadow(radius: 5)
.offset(x: 20, y: 20)
}
}
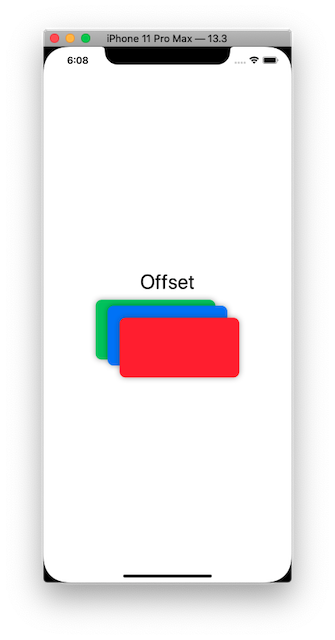
struct ContentView: View {
var body: some View {
VStack {
Text("Mask")
.font(.largeTitle)
Text("Mask is use to cut out background with other view ")
.padding()
.frame(maxWidth: .infinity)
.background(Color.orange)
Image("Flag-India")
.resizable()
.scaledToFill()
.frame(width: 100, height: 100)
.mask(Circle())
Image("images")
.resizable()
.frame(width: 100, height: 100)
.mask(
Text("INDIA")
.fontWeight(.black)
.font(.largeTitle)
)
Image("images")
.resizable()
.frame(width: 100, height: 100)
.mask(Image(systemName: "tortoise").resizable())
Spacer()
}
}
}
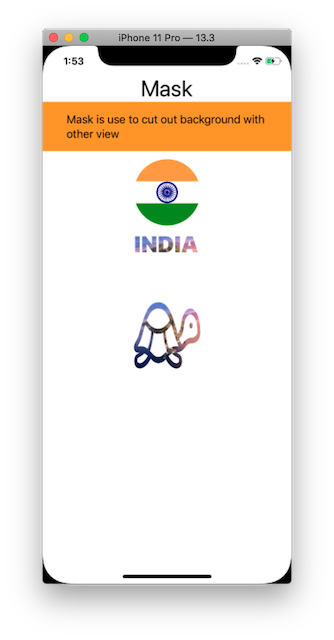
struct ContentView: View {
@State var degree:Double = 360
var body: some View {
VStack(spacing: 30) {
Text("Rotation")
.font(.largeTitle)
HStack {
Text("45")
.rotationEffect(Angle.degrees(45))
Text("-45")
.rotationEffect(Angle.degrees(-45))
Text("90")
.rotationEffect(Angle.degrees(90))
Text("180")
.rotationEffect(Angle.degrees(180))
Text("270")
.rotationEffect(Angle.degrees(270))
Text("360")
.rotationEffect(Angle.degrees(360))
}
Spacer()
Text("Rotation")
.font(.caption)
.padding()
.rotationEffect(Angle.degrees(degree))
.border(Color.orange)
HStack {
Text("Top Rotation")
.font(.caption)
.padding()
.rotationEffect(Angle.degrees(degree), anchor: .top)
.border(Color.orange)
Text("TopLeading Rotation")
.font(.caption)
.padding()
.rotationEffect(Angle.degrees(degree), anchor: .topLeading)
.border(Color.orange)
}
HStack {
Text("Bottom Rotation")
.font(.caption)
.padding()
.rotationEffect(Angle.degrees(degree), anchor: .bottom)
.border(Color.orange)
Text("BottomLeading Rotation")
.font(.caption)
.padding()
.rotationEffect(Angle.degrees(degree), anchor: .bottomLeading)
.border(Color.orange)
}
Slider(value: $degree, in: 0...360, step: 5.0)
.padding()
Text("\(degree, specifier: "%.2f") Degree")
Spacer()
}
}
}
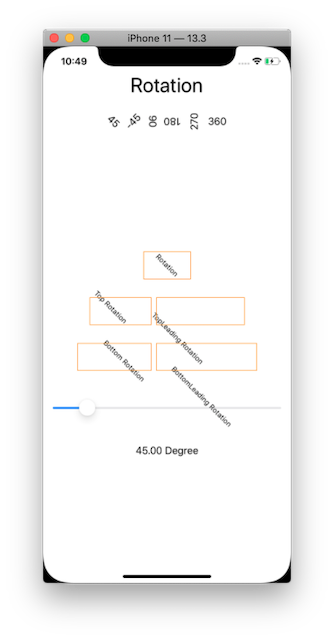