Status
Branch: reakit
I'm really impressed how this project, which started as something highly experimental, has evolved so much. And this is certainly due to the fact that so many people have believed on it, either collaborating directly or just testing it on their projects.
This project is one of the reasons I quit my job a few months ago. Improving it is my highest priority right now.
I'm opening this issue to tell you guys what I'm planning for Reas in the near future, to say thank you for collaborating on this project, and to invite you to participate in what's to come.
@Thomazella @Andarist @jyash97 @wordofchristian @joao-alberto @Thebigbignooby @boywithsilverwings @mgmgpyaesonewin @menor @nathanforce
ReaKit
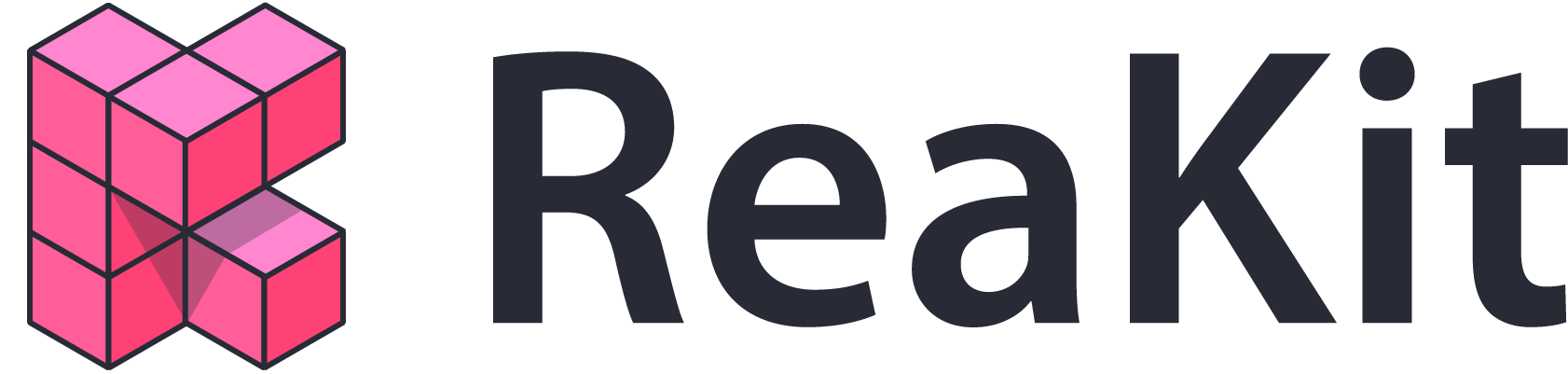
When I first designed Reas, its only focus was composability. as
was the main method responsible for ensuring high composability, and every component was built around it. So, the name of the library was an obvious combination of "React" and "as".
Let me give you a brief example of what I mean by composability. Let's create some styled components:
import { styled, Base, Button as BaseButton } from "reakit";
const Button = styled(BaseButton)`
text-transform: uppercase;
font-weight: 600;
`;
const ButtonRounded = styled(Button)`
border-radius: 1.25em;
padding: 0 1.375em;
`;
const ButtonPrimary = styled(Button)`
background-color: #fc4577;
border: none;
color: white;
`;
Now, we can render each one separately:
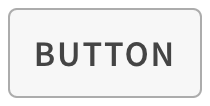
<ButtonRounded>Rounded</ButtonRounded>
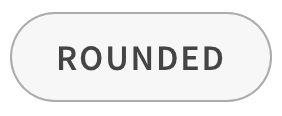
<ButtonPrimary>Primary</ButtonPrimary>
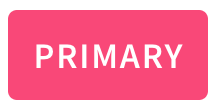
Finally, we can leverage composability and combine them:
<ButtonPrimary as={ButtonRounded}>Primary + Rounded</ButtonPrimary>
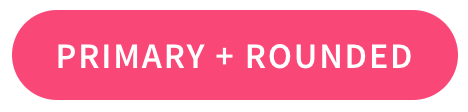
As the project evolved, it became more ambitious. Not only it needed to be composable, but also accessible, and a focus on interactivity was emerging with Containers. It turned out that calling it a "Toolkit" was more appropriate.
And, as you have noticed, I like the obvious:
React + Toolkit = ReaKit
Keeping the tradition of #40, I also don't know how to pronounce it. 😆
Changing the repository name should be seamless. Commit history and everything will be kept. GitHub will automatically redirect diegohaz/reas
to diegohaz/reakit
and the latter to reakit/reakit
.
Migrating to https://www.npmjs.com/package/reakit should be as easy as just replacing all instances of reas
to reakit
or creating an alias.
Thanks to @brunomacf and @marco-souza from @nosebit for the npm package name.
The new website is being built in the reakit branch and should be done by the next few weeks. Here are some screenshots of it:
The work on master
will continue as normal during this time. Nothing will change.
If you like what you read and would like to follow or contribute to this future, join us on https://reakit.slack.com (message me your email address and I'll send you an invite).
Thank you, guys.